FormControl
Enhance form usability with FormControl components in React. Manage validation, disabled states, and more. Easy integration for seamless form handling.
Installation
API Reference
This is an illustration of FormControl component.
size
isDisabled
isReadOnly
isRequired
import { Button, ButtonText } from "@/components/ui/button"import {FormControl,FormControlError,FormControlErrorText,FormControlErrorIcon,FormControlLabel,FormControlLabelText,FormControlHelper,FormControlHelperText,} from "@/components/ui/form-control"import { Input, InputField } from "@/components/ui/input"import { VStack } from "@/components/ui/vstack"import { AlertCircleIcon } from "@/components/ui/icon"import React from "react"function App() {const [isInvalid, setIsInvalid] = React.useState(false)const [inputValue, setInputValue] = React.useState("12345")const handleSubmit = () => {if (inputValue.length < 6) {setIsInvalid(true)} else {setIsInvalid(false)}}return (<VStack className="w-full max-w-[300px] rounded-md border border-background-200 p-4"><FormControlisInvalid={isInvalid}size="md"isDisabled={false}isReadOnly={false}isRequired={false}><FormControlLabel><FormControlLabelText>Password</FormControlLabelText></FormControlLabel><Input className="my-1" size={props.size}><InputFieldtype="password"placeholder="password"value={inputValue}onChangeText={(text) => setInputValue(text)}/></Input><FormControlHelper><FormControlHelperText>Must be atleast 6 characters.</FormControlHelperText></FormControlHelper><FormControlError><FormControlErrorIcon as={AlertCircleIcon} /><FormControlErrorText>Atleast 6 characters are required.</FormControlErrorText></FormControlError></FormControl><Button className="w-fit self-end mt-4" size="sm" onPress={handleSubmit}><ButtonText>Submit</ButtonText></Button></VStack>)}
Installation
CLI
Manual
Run the following command:
npx gluestack-ui add form-control
API Reference
To use this component in your project, include the following import statement in your file.
import { FormControl } from "@/components/ui/form-control"
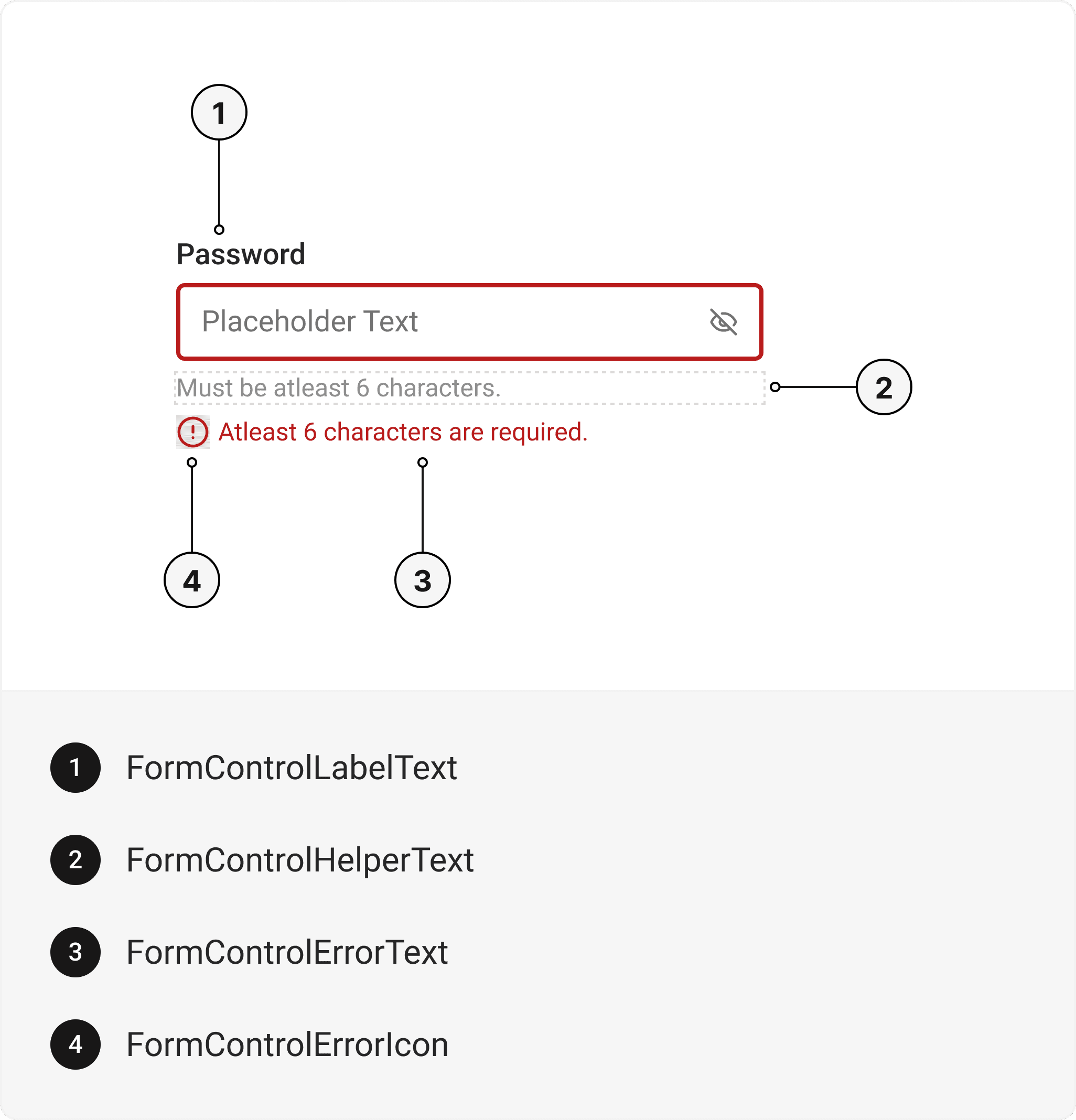
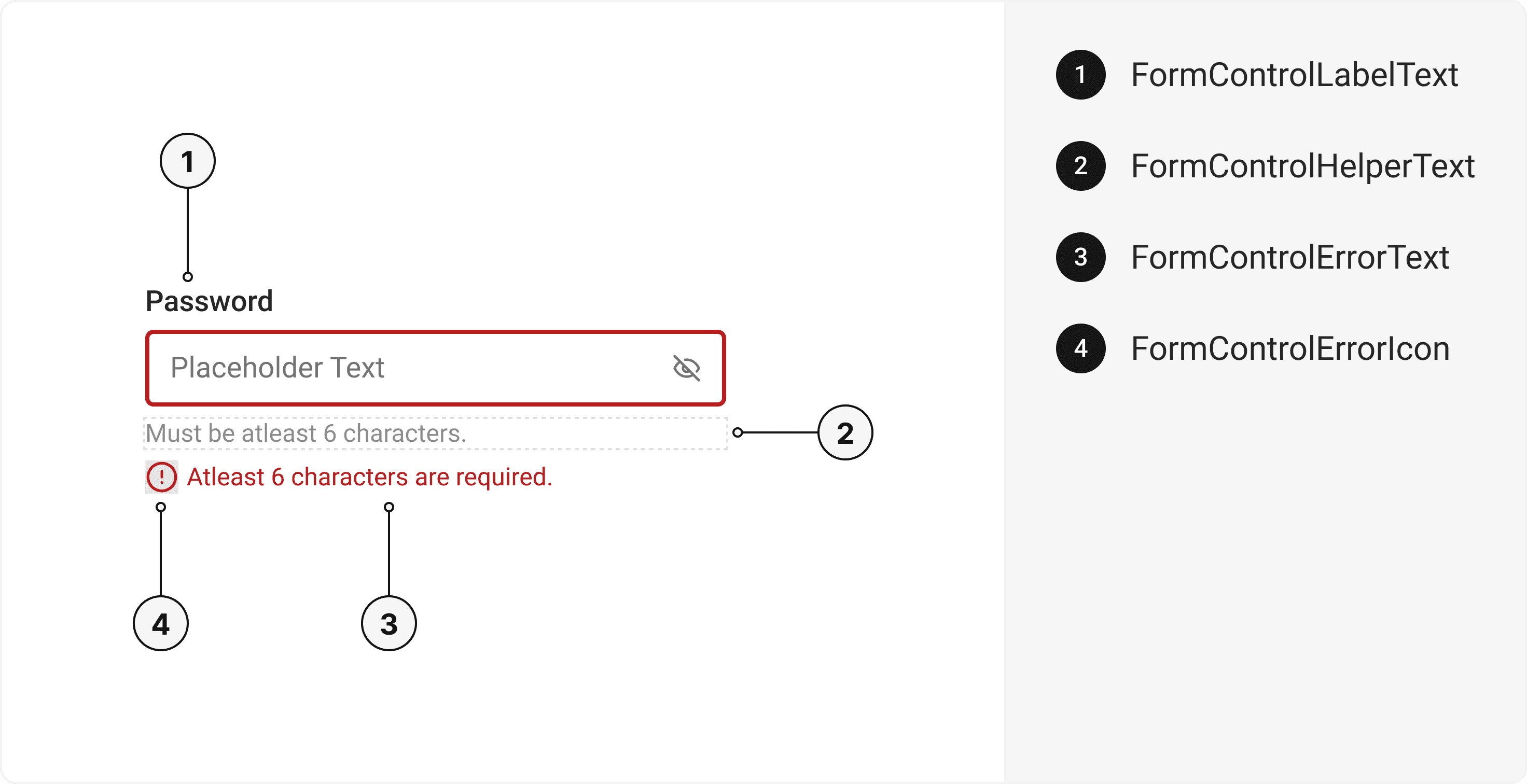
export default () => (<FormControl><FormControlLabel><FormControlLabelText /></FormControlLabel><FormControlHelper><FormControlHelperText /></FormControlHelper><FormControlError><FormControlErrorIcon /><FormControlErrorText /></FormControlError></FormControl>)
Component Props
This section provides a comprehensive reference list for the component props, detailing descriptions, properties, types, and default behavior for easy project integration.
FormControl
It inherits all the properties of React Native's View component.
Prop | Type | Default | Description |
---|---|---|---|
isInvalid | bool | false | When true, invalid state. |
isRequired | bool | false | If true, astrick gets activated. |
isDisabled | bool | false | Disabled state true. |
isReadOnly | bool | false | To manually set read-only state. |
isDisabled | bool | false | To manually set disable to the FormControl. |
FormControlLabel
It inherits all the properties of React Native's View component.
FormControlLabelText
It inherits all the properties of React Native's Text component.
FormControlHelper
It inherits all the properties of React Native's View component.
FormControlHelperText
It inherits all the properties of React Native's Text component.
FormControlError
It inherits all the properties of React Native's View component.
FormControlErrorIcon
It inherits all the properties of gluestack Style's AsForwarder component.
FormControlErrorText
It inherits all the properties of React Native's Text component.
Features
- Keyboard support for actions.
- Support for hover, focus and active states.
- Option to add your styles or use the default styles.
Props
FormControl component is created using View component from react-native. It extends all the props supported by React Native View.
Examples
The Examples section provides visual representations of the different variants of the component, allowing you to quickly and easily determine which one best fits your needs. Simply copy the code and integrate it into your project.
Form Control with Radio
The Radio Component can be incorporated within the FormControl.
import {FormControl,FormControlLabel,FormControlLabelText,FormControlHelper,FormControlHelperText,} from "@/components/ui/form-control"import {Radio,RadioGroup,RadioIndicator,RadioLabel,RadioIcon,} from "@/components/ui/radio"import { VStack } from "@/components/ui/vstack"import { CircleIcon } from "@/components/ui/icon"import React from "react"function App() {const [values, setValues] = React.useState("Mango")return (<FormControl><FormControlLabel><FormControlLabelText>Favourite fruit</FormControlLabelText></FormControlLabel><RadioGroup className="my-2" value={values} onChange={setValues}><VStack space="sm"><Radio size="sm" value="Mango"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Mango</RadioLabel></Radio><Radio size="sm" value="Apple"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Apple</RadioLabel></Radio><Radio size="sm" value="Orange"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Orange</RadioLabel></Radio></VStack></RadioGroup><FormControlHelper><FormControlHelperText>Choose the fruit you like the most</FormControlHelperText></FormControlHelper></FormControl>)}
Form Control with Checkbox
The Checkbox Component can be incorporated within the FormControl.
import {Checkbox,CheckboxIndicator,CheckboxLabel,CheckboxIcon,CheckboxGroup,} from "@/components/ui/checkbox"import {FormControl,FormControlLabel,FormControlLabelText,FormControlHelper,FormControlHelperText,} from "@/components/ui/form-control"import { VStack } from "@/components/ui/vstack"import { CheckIcon } from "@/components/ui/icon"import React from "react"function App() {const [values, setValues] = React.useState(["bits"])return (<FormControl><FormControlLabel><FormControlLabelText>Sign up for newsletters</FormControlLabelText></FormControlLabel><CheckboxGroupclassName="my-2"value={values}onChange={(keys) => {setValues(keys)}}><VStack space="sm"><Checkbox size="sm" value="bits"><CheckboxIndicator className="mr-2"><CheckboxIcon as={CheckIcon} /></CheckboxIndicator><CheckboxLabel>Daily Bits</CheckboxLabel></Checkbox><Checkbox size="sm" value="event"><CheckboxIndicator className="mr-2"><CheckboxIcon as={CheckIcon} /></CheckboxIndicator><CheckboxLabel>Event Updates</CheckboxLabel></Checkbox><Checkbox size="sm" value="sponsorship"><CheckboxIndicator className="mr-2"><CheckboxIcon as={CheckIcon} /></CheckboxIndicator><CheckboxLabel>Sponsorship</CheckboxLabel></Checkbox></VStack></CheckboxGroup><FormControlHelper><FormControlHelperText>Subscribe to newsletters for updates</FormControlHelperText></FormControlHelper></FormControl>)}
Form Control with Textarea
The Textarea Component can be incorporated within the FormControl.
import {FormControl,FormControlLabel,FormControlLabelText,FormControlHelper,FormControlHelperText,} from "@/components/ui/form-control"import { Textarea, TextareaInput } from "@/components/ui/textarea"function Example() {return (<FormControl><FormControlLabel><FormControlLabelText>Comment</FormControlLabelText></FormControlLabel><Textarea className="min-w-[200px]"><TextareaInput /></Textarea><FormControlHelper><FormControlHelperText>Type your comment above</FormControlHelperText></FormControlHelper></FormControl>)}
Form Control with Error
Error messages can be displayed using FormControl.
import {FormControl,FormControlError,FormControlErrorText,FormControlErrorIcon,FormControlLabel,FormControlLabelText,} from "@/components/ui/form-control"import {Radio,RadioGroup,RadioIndicator,RadioLabel,RadioIcon,} from "@/components/ui/radio"import { VStack } from "@/components/ui/vstack"import { AlertCircleIcon, CircleIcon } from "@/components/ui/icon"function Example() {return (<FormControl isInvalid><FormControlLabel><FormControlLabelText>Which time slot works best for you?</FormControlLabelText></FormControlLabel><RadioGroup className="my-2"><VStack space="sm"><Radio size="sm" value="Mango"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Monday</RadioLabel></Radio><Radio size="sm" value="Apple"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Tuesday</RadioLabel></Radio><Radio size="sm" value="Orange"><RadioIndicator><RadioIcon as={CircleIcon} /></RadioIndicator><RadioLabel>Wednesday</RadioLabel></Radio></VStack></RadioGroup><FormControlError><FormControlErrorIcon as={AlertCircleIcon} /><FormControlErrorText>Choose one time slot for the meeting</FormControlErrorText></FormControlError></FormControl>)}
Form Control with Form Actions
Form Action Buttons can also be utilized in conjunction with FormControl.
import { Button, ButtonText } from "@/components/ui/button"import { FormControl } from "@/components/ui/form-control"import { HStack } from "@/components/ui/hstack"function Example() {return (<HStack><FormControl><Button variant="outline" action="secondary"><ButtonText>Cancel</ButtonText></Button></FormControl><FormControl><Button action="negative" className="ml-4"><ButtonText className="text-white group-hover/button:text-white group-active/button:text-white">Delete</ButtonText></Button></FormControl></HStack>)}