Menu
Build a user-friendly interface with gluestack-ui menu component in React & React Native, designed for easy navigation and accessibility.
Installation
API Reference
This is an illustration of a Menu component.
placement
import { Button, ButtonText } from "@/components/ui/button"import { Menu, MenuItem, MenuItemLabel } from "@/components/ui/menu"import {Icon,AddIcon,GlobeIcon,PlayIcon,SettingsIcon,} from "@/components/ui/icon"function Example() {return (<Menuplacement="top"offset={5}disabledKeys={["Settings"]}trigger={({ ...triggerProps }) => {return (<Button {...triggerProps}><ButtonText>Menu</ButtonText></Button>)}}><MenuItem key="Add account" textValue="Add account"><Icon as={AddIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Add account</MenuItemLabel></MenuItem><MenuItem key="Community" textValue="Community"><Icon as={GlobeIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Community</MenuItemLabel></MenuItem><MenuItem key="Plugins" textValue="Plugins"><Icon as={PlayIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Plugins</MenuItemLabel></MenuItem><MenuItem key="Settings" textValue="Settings"><Icon as={SettingsIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Settings</MenuItemLabel></MenuItem></Menu>)}
Installation
CLI
Manual
Run the following command:
npx gluestack-ui add menu
API Reference
To use this component in your project, include the following import statement in your file.
import {Menu,MenuItem,MenuItemLabel,MenuSeparator,} from "@/components/ui/menu"
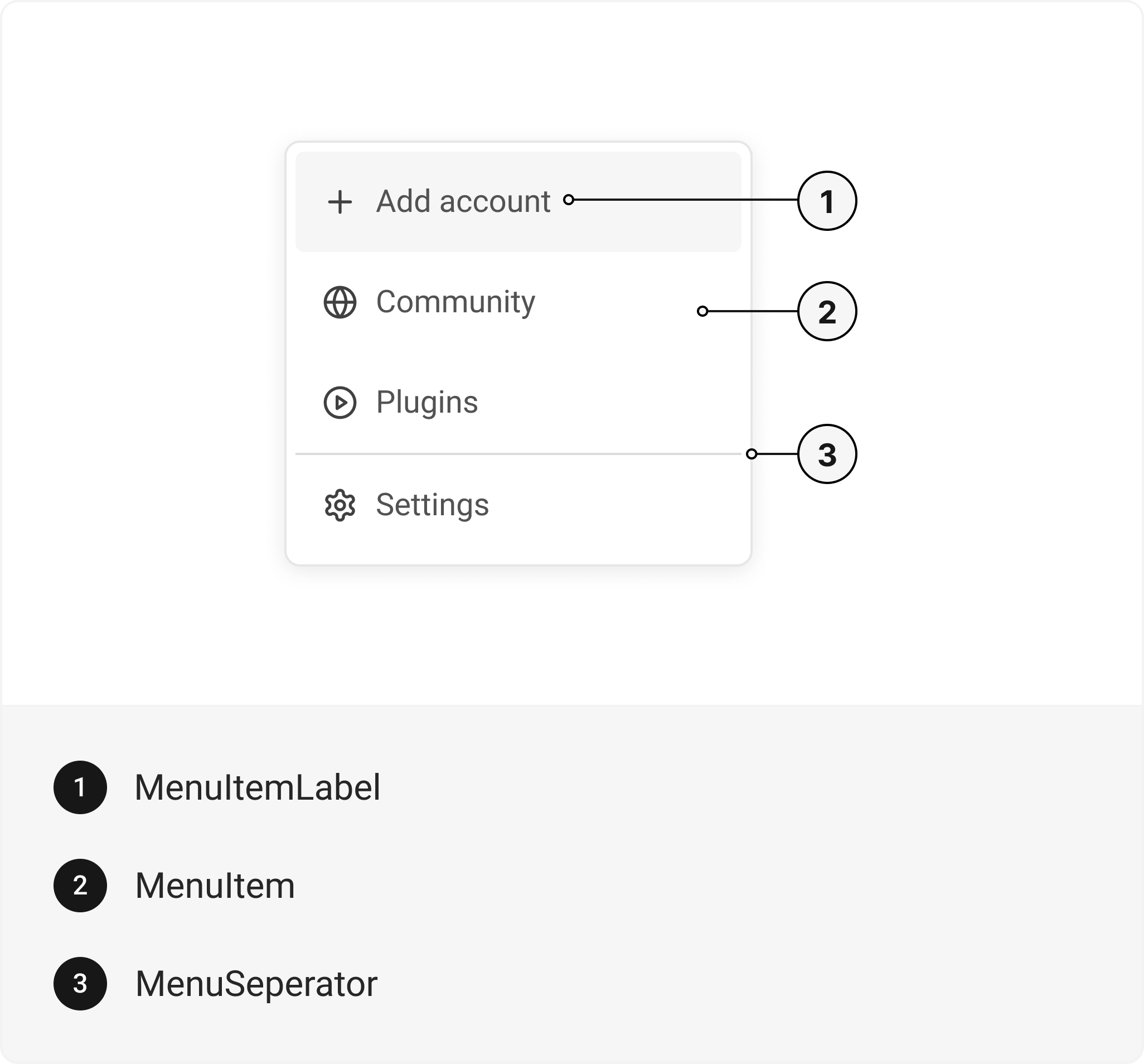
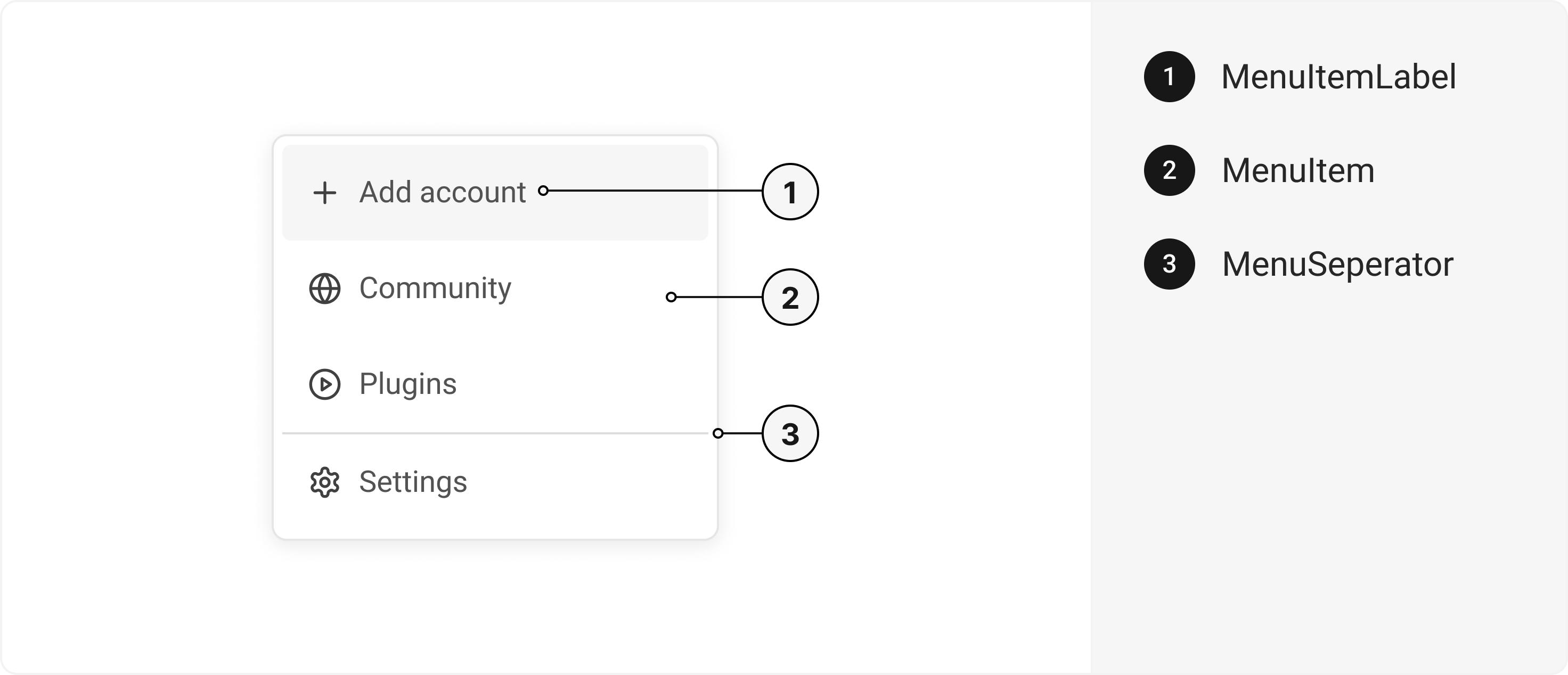
export default () => (<Menu><MenuItem><MenuItemLabel /></MenuItem><MenuSeparator /></Menu>)
Important Note
Note: The immediate parent of MenuItem must be Menu. There should be no higher-order component (HOC) between them.
Component Props
This section provides a comprehensive reference list for the component props, detailing descriptions, properties, types, and default behavior for easy project integration.
Menu
Contains all menu related layout style props and actions.
It inherits all the properties of React Native's View component.
Prop | Type | Default | Description |
---|---|---|---|
trigger | (_props: any, state: { open: boolean; }) => Element | - | Function that returns a React Element. This element will be used as a Trigger for the Menu |
placement | "bottom" | "top" | "right" | "left" | "top left" | "top right" | "bottom left" | "bottom right" | "right top" | "right bottom" | "left top" | "left bottom" | bottom left | menu placement |
defaultIsOpen | boolean | false | If true, the menu will be opened by default. |
onOpen | () => void | true | This function will be invoked when the menu is opened. |
onClose | () => void | - | This function will be invoked when menu is closed. It will also be called when the user attempts to close the menu via Escape key or backdrop press. |
isOpen | boolean | false | Whether the menu is opened. Useful for controlling the open state. |
offset | number | - | The additional offset applied along the main axis between the
element and its trigger element. |
crossOffset | number | - | The additional offset applied along the cross axis between the
element and its trigger element. |
disabledKeys | string [] | - | Item keys in this collection will be disabled. |
closeOnSelect | boolean | true | This prop determine whether menu is closed after option is selected. |
selectedKeys | 'all' | Iterable<Key> | - | The currently selected keys in the collection (controlled). |
selectionMode | 'none'| 'single' | 'multiple' | - | The type of selection that is allowed in the collection. |
onSelectionChange | (keys: 'all' | Iterable<Key>) => void | - | Handler that is called when the selection changes. |
MenuItem
Contains all button related layout style props and actions. It inherits all the properties of React Native's Pressable component.
Prop | Type | Default | Description |
---|---|---|---|
closeOnSelect | boolean | true | This prop determine whether menu is closed after option is selected. |
MenuItemLabel
Contains all text related layout style props and actions. It inherits all the properties of React Native's Text component.
MenuSeparator
Contains all view related layout style props and actions. It inherits all the properties of React Native's View component.
Features
- Keyboard support for actions.
- Support for hover, focus and active states.
Accessibility
We have outlined the various features that ensure the Menu component is accessible to all users, including those with disabilities. These features help ensure that your application is inclusive and meets accessibility standards. Adheres to the MENU WAI-ARIA design pattern.
Keyboard
- Space: Opens/closes the menu.
- Enter: Opens/closes the menu.
- Arrow Down: Moves focus to the next focusable element.
- Arrow Up: Moves focus to the previous focusable element.
- Esc: Closes the menu and moves focus to menuTrigger.
Screen Reader
- VoiceOver: When the menu is opened, the screen reader will say, Menu item group with 4 items. You are currently on a menu item group. To select this menu item, press Control + Option + Space. To close the menu, press Escape.
States
- aria-labelledby identifies the element that labels the current element.
- aria-label defines a string value that labels the current element.
Examples
The Examples section provides visual representations of the different variants of the component, allowing you to quickly and easily determine which one best fits your needs. Simply copy the code and integrate it into your project.
Menu with Tag
import { Badge, BadgeText } from "@/components/ui/badge"import { Button, ButtonIcon } from "@/components/ui/button"import {Menu,MenuItem,MenuItemLabel,MenuSeparator,} from "@/components/ui/menu"import { MenuIcon } from "@/components/ui/icon"function Example() {return (<Menuoffset={5}trigger={({ ...triggerProps }) => {return (<Button {...triggerProps} size="sm"><ButtonIcon as={MenuIcon} /></Button>)}}><MenuItemkey="Membership"textValue="Membership"className="p-2 justify-between"><MenuItemLabel size="sm">Membership</MenuItemLabel><Badge action="success" className="rounded-full"><BadgeText className="text-2xs capitalize">Pro</BadgeText></Badge></MenuItem><MenuItem key="Orders" textValue="Orders" className="p-2"><MenuItemLabel size="sm">Orders</MenuItemLabel></MenuItem><MenuItem key="Address Book" textValue="Address Book" className="p-2"><MenuItemLabel size="sm">Address Book</MenuItemLabel></MenuItem><MenuSeparator /><MenuItem key="Earn & Redeem" textValue="Earn & Redeem" className="p-2"><MenuItemLabel size="sm">Earn & Redeem</MenuItemLabel></MenuItem><MenuItem key="Coupons" textValue="Coupons" className="p-2"><MenuItemLabel size="sm">Coupons</MenuItemLabel></MenuItem><MenuItem key="Help Center" textValue="Help Center" className="p-2"><MenuItemLabel size="sm">Help Center</MenuItemLabel></MenuItem><MenuSeparator /><MenuItem key="Logout" textValue="Logout" className="p-2"><MenuItemLabel size="sm">Logout</MenuItemLabel></MenuItem></Menu>)}
Menu with Selection
import { Button, ButtonText } from "@/components/ui/button"import {Menu,MenuItem,MenuItemLabel,MenuSeparator,} from "@/components/ui/menu"import {Icon,HelpCircleIcon,MessageCircleIcon,SettingsIcon,} from "@/components/ui/icon"import React from "react"function Example() {const [selected, setSelected] = React.useState(new Set([]))return (<Menuplacement="bottom left"selectionMode="single"selectedKeys={selected}offset={5}className="p-1.5"onSelectionChange={(keys) => {setSelected(keys)if (keys.currentKey === "Account Setting") {console.log("Push to", keys.currentKey, "route")} else if (keys.currentKey === "Help Centre") {console.log("Push to", keys.currentKey, "route")} else if (keys.currentKey === "Contact Support") {console.log("Push to", keys.currentKey, "route")} else if (keys.currentKey === "Download Mobile App") {console.log("Push to", keys.currentKey, "route")} else if (keys.currentKey === "Install Chrome Extension") {console.log("Push to", keys.currentKey, "route")}}}closeOnSelect={true}trigger={({ ...triggerProps }) => {return (<Button {...triggerProps}><ButtonText>Menu</ButtonText></Button>)}}><MenuItemkey="Account Settings"textValue="Account Settings"className="p-2 web:min-w-[294px] min-w-[225px]"><Icon as={SettingsIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Account Settings</MenuItemLabel></MenuItem><MenuItem key="Help Centre" textValue="Help Centre" className="p-2"><Icon as={HelpCircleIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Help Centre</MenuItemLabel></MenuItem><MenuItemkey="Contact Support"textValue="Contact Support"className="p-2"><Icon as={MessageCircleIcon} size="sm" className="mr-2" /><MenuItemLabel size="sm">Contact Support</MenuItemLabel></MenuItem><MenuSeparator /><MenuItemkey="Download Mobile App"textValue="Download Mobile App"className="p-2"><MenuItemLabel size="sm">Download Mobile App</MenuItemLabel></MenuItem><MenuItemkey="Install Chrome Extension"textValue="Install Chrome Extension"className="p-2"><MenuItemLabel size="sm">Install Chrome Extension</MenuItemLabel></MenuItem></Menu>)}