Modal
Create smooth and accessible modals in React & React Native. Implement React modal components for alerts, forms, and notifications with ease. Optimize modal component for better user engagement.
Installation
API Reference
This is an illustration of Modal component.
size
import { Button, ButtonText } from "@/components/ui/button"import { Center } from "@/components/ui/center"import { Heading } from "@/components/ui/heading"import {Modal,ModalBackdrop,ModalContent,ModalCloseButton,ModalHeader,ModalBody,ModalFooter,} from "@/components/ui/modal"import { Text } from "@/components/ui/text"import { Icon, CloseIcon } from "@/components/ui/icon"import React from "react"function App() {const [showModal, setShowModal] = React.useState(false)return (<Center className="h-[300px]"><Button onPress={() => setShowModal(true)}><ButtonText>Show Modal</ButtonText></Button><ModalisOpen={showModal}onClose={() => {setShowModal(false)}}size="md"><ModalBackdrop /><ModalContent><ModalHeader><Heading size="md" className="text-typography-950">Invite your team</Heading><ModalCloseButton><Iconas={CloseIcon}size="md"className="stroke-background-400 group-[:hover]/modal-close-button:stroke-background-700 group-[:active]/modal-close-button:stroke-background-900 group-[:focus-visible]/modal-close-button:stroke-background-900"/></ModalCloseButton></ModalHeader><ModalBody><Text size="sm" className="text-typography-500">Elevate user interactions with our versatile modals. Seamlesslyintegrate notifications, forms, and media displays. Make an impacteffortlessly.</Text></ModalBody><ModalFooter><Buttonvariant="outline"action="secondary"onPress={() => {setShowModal(false)}}><ButtonText>Cancel</ButtonText></Button><ButtononPress={() => {setShowModal(false)}}><ButtonText>Explore</ButtonText></Button></ModalFooter></ModalContent></Modal></Center>)}
Installation
CLI
Manual
Run the following command:
npx gluestack-ui add modal
API Reference
To use this component in your project, include the following import statement in your file.
import {Modal,ModalBackdrop,ModalContent,ModalHeader,ModalCloseButton,ModalBody,ModalFooter,} from "@/components/ui/modal"
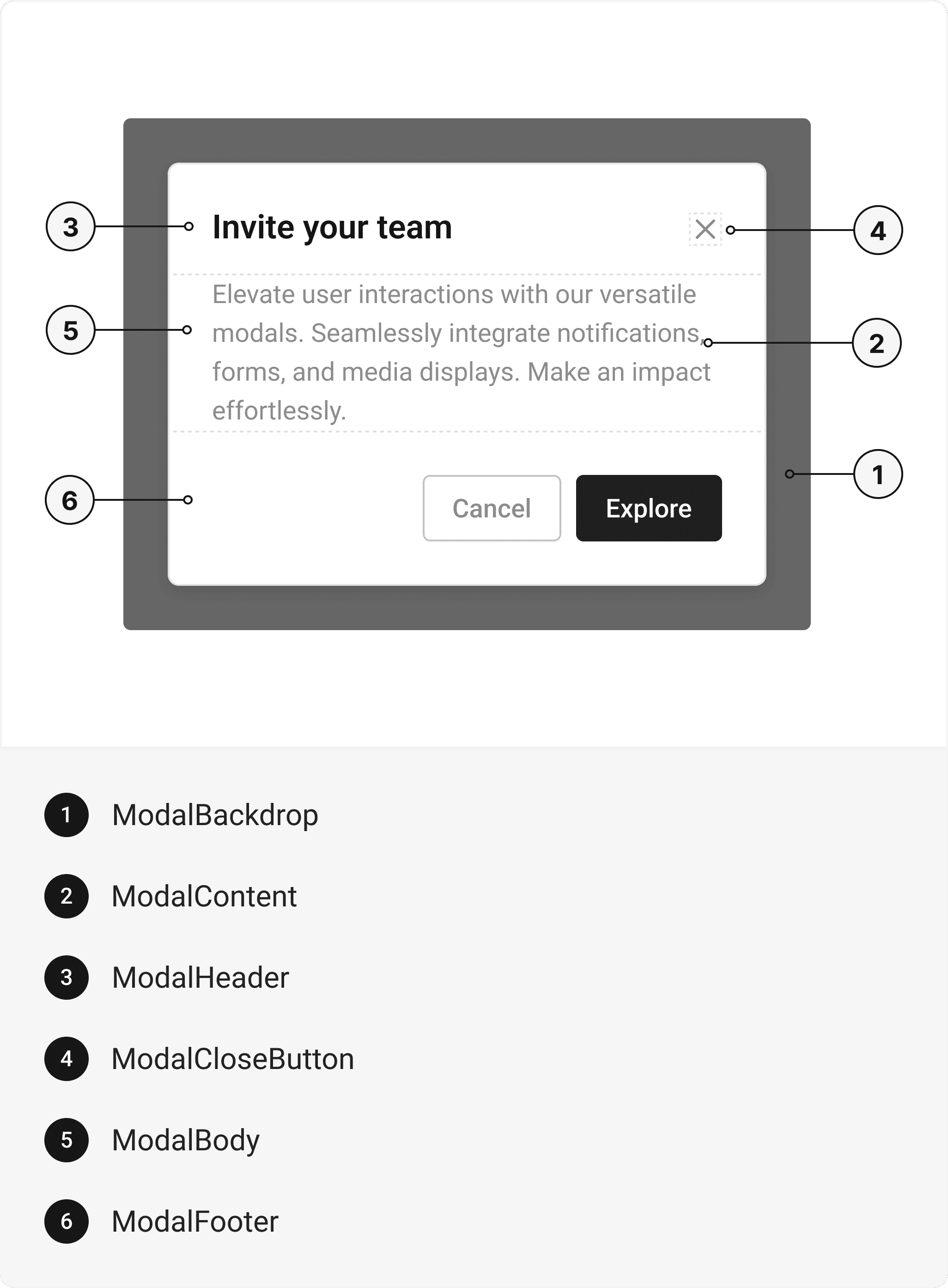
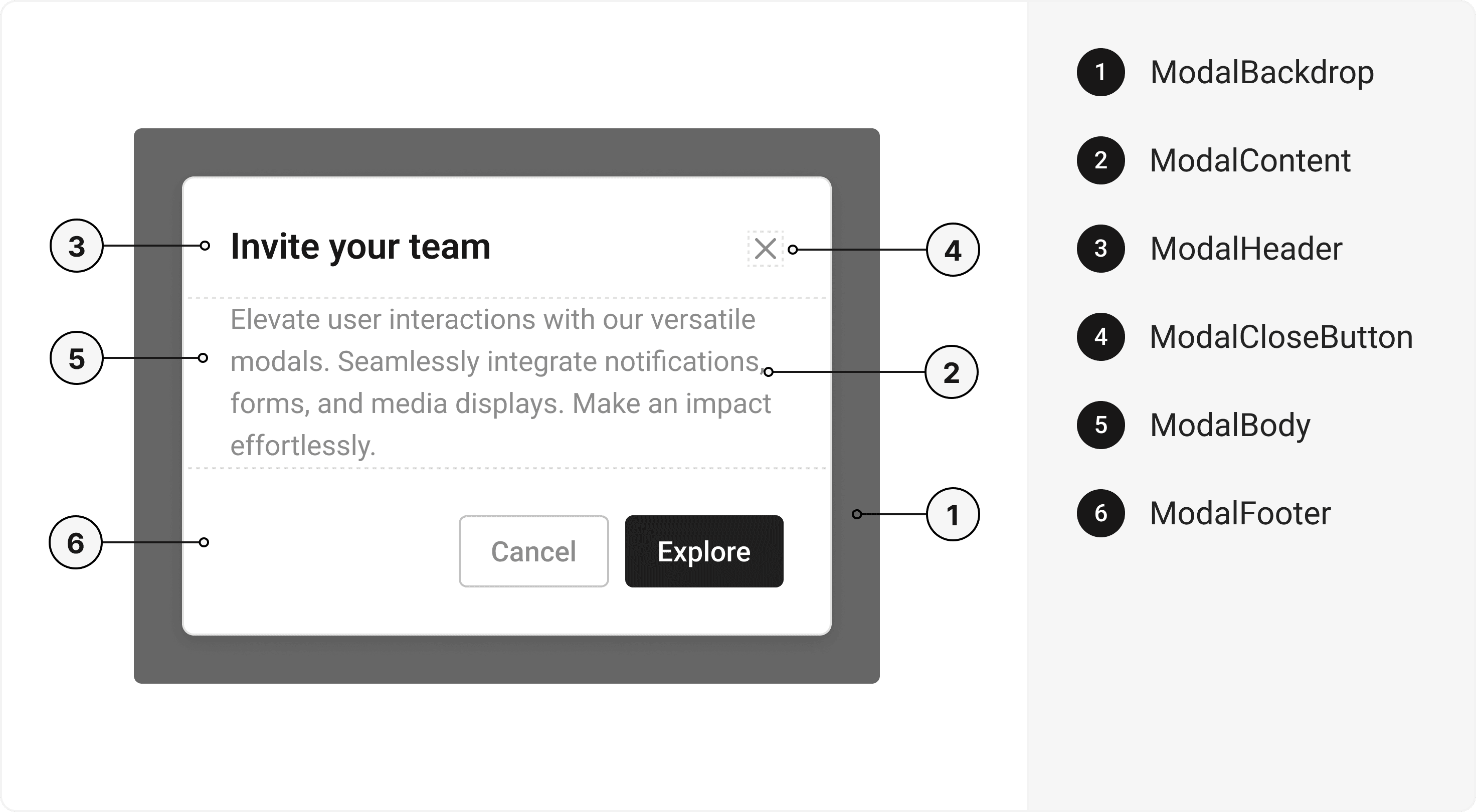
export default () => (<Modal><ModalBackdrop /><ModalContent><ModalHeader><ModalCloseButton></ModalCloseButton></ModalHeader><ModalBody /><ModalFooter /></ModalContent></Modal>)
Component Props
This section provides a comprehensive reference list for the component props, detailing descriptions, properties, types, and default behavior for easy project integration.
Modal
Contains all View related layout style props and actions. It inherits all the properties of React Native's View component.
Prop | Type | Default | Description |
---|---|---|---|
isOpen | boolean | - | If true, the modal will open. Useful for controllable state behavior. |
onClose | () => any | - | Callback invoked when the modal is closed. |
useRNModal | boolean | false | If true, renders react-native native modal. |
defaultIsOpen | boolean | - | Specifies the default open state of the Modal |
initialFocusRef | React.RefObject<any> | - | The ref of element to receive focus when the modal opens. |
finalFocusRef | React.RefObject<any> | - | The ref of element to receive focus when the modal closes |
avoidKeyboard | boolean | - | If true, the Modal will avoid the keyboard. |
closeOnOverlayClick | boolean | - | If true, the Modal will close when the overlay is clicked. |
isKeyboardDismissable | boolean | - | If true, the keyboard can dismiss the Modal |
children | any | - | The content to display inside the Modal |
ModalBackdrop
It is React Native's Pressable component, created using @legendapp/motion's createMotionAnimatedComponent function to add animation to the component. You can use any declarative animation library you prefer.
ModalContent
It is @legendapp/motion's Motion.View component. You can use any declarative animation library you prefer.
Prop | Type | Default | Description |
---|---|---|---|
focusable | boolean | false | If true, Modal Content will be focusable. |
ModalHeader
It inherits all the properties of React Native's View component.
ModalCloseButton
It inherits all the properties of React Native's View component.
ModalBody
It inherits all the properties of React Native's View component
ModalFooter
It inherits all the properties of React Native's View component.
Accessibility
We have outlined the various features that ensure the Modal component is accessible to all users, including those with disabilities. These features help ensure that your application is inclusive and meets accessibility standards. It uses React Native ARIA @react-native-aria/focus which follows the Dialog Modal WAI-ARIA design pattern.
Props
Modal component is created using View component from react-native. It extends all the props supported by React Native View, utility props and the props mentioned below.
Modal
Name | Value | Default |
---|---|---|
size | xs | sm | md | lg | full | md |
Examples
The Examples section provides visual representations of the different variants of the component, allowing you to quickly and easily determine which one best fits your needs. Simply copy the code and integrate it into your project.
Multiple Modals
A user interface featuring multiple modal components that allow for layered interaction and context-specific information display.
import { Button, ButtonText, ButtonIcon } from "@/components/ui/button"import { Heading } from "@/components/ui/heading"import { HStack } from "@/components/ui/hstack"import { Input, InputField } from "@/components/ui/input"import { Link, LinkText } from "@/components/ui/link"import {Modal,ModalBackdrop,ModalContent,ModalHeader,ModalBody,ModalFooter,} from "@/components/ui/modal"import { Text } from "@/components/ui/text"import { ArrowLeftIcon } from "@/components/ui/icon"import React from "react"function App() {const [showModal, setShowModal] = React.useState(false)const [showModal2, setShowModal2] = React.useState(false)const [showModal3, setShowModal3] = React.useState(false)return (<><Button onPress={() => setShowModal(true)}><ButtonText>Forgot password?</ButtonText></Button><ModalisOpen={showModal}onClose={() => {setShowModal(false)}}><ModalBackdrop /><ModalContent><ModalHeader className="flex-col items-start gap-0.5"><Heading>Forgot password?</Heading><Text size="sm">No worries, we’ll send you reset instructions</Text></ModalHeader><ModalBody className="mb-4"><Input><InputField placeholder="Enter your email" /></Input></ModalBody><ModalFooter className="flex-col items-start"><ButtononPress={() => {setShowModal2(true)}}className="w-full"><ButtonText>Submit</ButtonText></Button><Buttonvariant="link"size="sm"onPress={() => {setShowModal(false)}}className="gap-1"><ButtonIcon as={ArrowLeftIcon} /><ButtonText>Back to login</ButtonText></Button></ModalFooter></ModalContent></Modal><ModalisOpen={showModal2}onClose={() => {setShowModal2(false)}}><ModalBackdrop /><ModalContent><ModalHeader className="flex-col items-start gap-0.5"><Heading>Reset password</Heading><Text size="sm">A verification code has been sent to you. Enter code below.</Text></ModalHeader><ModalBody className="mb-4"><Input><InputField placeholder="Enter verification code" /></Input></ModalBody><ModalFooter className="flex-col items-start"><ButtononPress={() => {setShowModal3(true)}}className="w-full"><ButtonText>Continue</ButtonText></Button><Text size="sm" className="">Didn’t receive the email?<Link className=""><LinkTextsize="xs"className="text-typography-700 font-semibold">Click to resend</LinkText></Link></Text><HStack space="xs" className="items-center"><Buttonvariant="link"size="sm"onPress={() => {setShowModal2(false)}}className="gap-1"><ButtonIcon as={ArrowLeftIcon} /><ButtonText>Back to login</ButtonText></Button></HStack></ModalFooter></ModalContent></Modal><ModalisOpen={showModal3}onClose={() => {setShowModal3(false)}}><ModalBackdrop /><ModalContent><ModalHeader className="flex-col items-start gap-0.5"><Heading>Set new password</Heading><Text size="sm">Almost done. Enter your new password and you are all set.</Text></ModalHeader><ModalBody className="" contentContainerClassName="gap-3"><Input><InputField placeholder="New password" /></Input><Input><InputField placeholder="Confirm new password" /></Input></ModalBody><ModalFooter className="flex-col items-start"><ButtononPress={() => {setShowModal(false)setShowModal2(false)setShowModal3(false)}}className="w-full"><ButtonText>Submit</ButtonText></Button><Buttonvariant="link"size="sm"onPress={() => {setShowModal3(false)}}className="gap-1"><ButtonIcon as={ArrowLeftIcon} /><ButtonText>Back to login</ButtonText></Button></ModalFooter></ModalContent></Modal></>)}
Onboarding Message
import { Button, ButtonText } from "@/components/ui/button"import { Heading } from "@/components/ui/heading"import { Image } from "@/components/ui/image"import {Modal,ModalBackdrop,ModalContent,ModalBody,ModalFooter,} from "@/components/ui/modal"import { Text } from "@/components/ui/text"import React from "react"function App() {const [showModal, setShowModal] = React.useState(false)return (<><Button onPress={() => setShowModal(true)}><ButtonText>Dashboard</ButtonText></Button><ModalisOpen={showModal}onClose={() => {setShowModal(false)}}><ModalBackdrop /><ModalContent className="max-w-[375px]"><Imagesource={{uri: "https://gluestack.github.io/public-blog-video-assets/Image%20Element.png",}}alt="image"className="h-[185px] w-full rounded"/><ModalBody className="mb-5" contentContainerClassName=""><Heading size="md" className="text-typography-950 text-center">Welcome to the dashboard</Heading><Text size="sm" className="text-typography-500 text-center">We are glad to have you on board, Here are some quick tips to letyou up and running.</Text></ModalBody><ModalFooter className="w-full"><Buttonvariant="outline"action="secondary"size="sm"onPress={() => {setShowModal(false)}}className="flex-grow"><ButtonText>Skip</ButtonText></Button><ButtononPress={() => {setShowModal(false)}}size="sm"className="flex-grow"><ButtonText>Next</ButtonText></Button></ModalFooter></ModalContent></Modal></>)}
Delete Post
import { Box } from "@/components/ui/box"import { Button, ButtonText } from "@/components/ui/button"import { Heading } from "@/components/ui/heading"import {Modal,ModalBackdrop,ModalContent,ModalHeader,ModalBody,ModalFooter,} from "@/components/ui/modal"import { Text } from "@/components/ui/text"import { Icon, TrashIcon } from "@/components/ui/icon"import React from "react"function App() {const [showModal, setShowModal] = React.useState(false)return (<><Button onPress={() => setShowModal(true)}><ButtonText>Delete Post</ButtonText></Button><ModalisOpen={showModal}onClose={() => {setShowModal(false)}}><ModalBackdrop /><ModalContent className="max-w-[305px] items-center"><ModalHeader><Box className="w-[56px] h-[56px] rounded-full bg-background-error items-center justify-center"><Icon as={TrashIcon} className="stroke-error-600" size="xl" /></Box></ModalHeader><ModalBody className="mt-0 mb-4"><Heading size="md" className="text-typography-950 mb-2 text-center">Delete blog post</Heading><Text size="sm" className="text-typography-500 text-center">Are you sure you want to delete this post? This action cannot beundone.</Text></ModalBody><ModalFooter className="w-full"><Buttonvariant="outline"action="secondary"size="sm"onPress={() => {setShowModal(false)}}className="flex-grow"><ButtonText>Cancel</ButtonText></Button><ButtononPress={() => {setShowModal(false)}}size="sm"className="flex-grow"><ButtonText>Delete</ButtonText></Button></ModalFooter></ModalContent></Modal></>)}
Invite Friends to Project
import { Button, ButtonText } from "@/components/ui/button"import { Heading } from "@/components/ui/heading"import { Input, InputField } from "@/components/ui/input"import {Modal,ModalBackdrop,ModalContent,ModalCloseButton,ModalHeader,ModalBody,} from "@/components/ui/modal"import { Pressable } from "@/components/ui/pressable"import { Text } from "@/components/ui/text"import { VStack } from "@/components/ui/vstack"import { Icon, CloseIcon, CopyIcon } from "@/components/ui/icon"import React from "react"function App() {const [showModal, setShowModal] = React.useState(false)return (<><Button onPress={() => setShowModal(true)}><ButtonText>Invite</ButtonText></Button><ModalisOpen={showModal}onClose={() => {setShowModal(false)}}><ModalBackdrop /><ModalContent className="max-w-[395px]"><ModalHeader className="gap-2 items-start"><VStack className="gap-1"><Heading size="md" className="text-typography-950">Invite your team</Heading><Text size="sm" className="text-typography-500">You have created a new project! Invite colleagues to collaborateon this project.</Text></VStack><ModalCloseButton><Icon as={CloseIcon} className="stroke-background-500" /></ModalCloseButton></ModalHeader><ModalBodyclassName="mb-0"contentContainerClassName="gap-4 space-between flex-row items-center"><Input variant="outline" size="sm" className="flex-1"><InputField placeholder="join.untitledui.com/personalproject" /></Input><Pressable className="h-9 w-9 justify-center items-center border border-outline-300 rounded"><Icon as={CopyIcon} className="stroke-background-800" /></Pressable></ModalBody></ModalContent></Modal></>)}